Add `showRemoveItemLink` filter to `CartLineItemRow` (https://github.com/woocommerce/woocommerce-blocks/pull/7242)
* Add showRemoveItemLink filter This is a filter that will allow developers to set whether the link to remove a cartItem is visible * Add test for showRemoveItemLink filter * Remove unnecessary quantity override * Move definition of cart to avoid hardcoding id value in filter * Updated documentation for `showRemoveItemLink` filter * available-filters.md tweak * available-filters.md tweak * Add example to filters for showRemoveItemLink * Remove whitespace Co-authored-by: Paulo Arromba <17236129+wavvves@users.noreply.github.com> Co-authored-by: Niels Lange <info@nielslange.de>
This commit is contained in:
parent
dcde8dee05
commit
2e13856173
|
@ -195,6 +195,13 @@ const CartLineItemRow = forwardRef< HTMLTableRowElement, CartLineItemRowProps >(
|
|||
validation: productPriceValidation,
|
||||
} );
|
||||
|
||||
const showRemoveItemLink = __experimentalApplyCheckoutFilter( {
|
||||
filterName: 'showRemoveItemLink',
|
||||
defaultValue: true,
|
||||
extensions,
|
||||
arg,
|
||||
} );
|
||||
|
||||
return (
|
||||
<tr
|
||||
className={ classnames(
|
||||
|
@ -301,33 +308,38 @@ const CartLineItemRow = forwardRef< HTMLTableRowElement, CartLineItemRowProps >(
|
|||
itemName={ name }
|
||||
/>
|
||||
) }
|
||||
<button
|
||||
className="wc-block-cart-item__remove-link"
|
||||
onClick={ () => {
|
||||
onRemove();
|
||||
removeItem();
|
||||
dispatchStoreEvent( 'cart-remove-item', {
|
||||
product: lineItem,
|
||||
quantity,
|
||||
} );
|
||||
speak(
|
||||
sprintf(
|
||||
/* translators: %s refers to the item name in the cart. */
|
||||
__(
|
||||
'%s has been removed from your cart.',
|
||||
'woo-gutenberg-products-block'
|
||||
),
|
||||
name
|
||||
)
|
||||
);
|
||||
} }
|
||||
disabled={ isPendingDelete }
|
||||
>
|
||||
{ __(
|
||||
'Remove item',
|
||||
'woo-gutenberg-products-block'
|
||||
) }
|
||||
</button>
|
||||
{ showRemoveItemLink && (
|
||||
<button
|
||||
className="wc-block-cart-item__remove-link"
|
||||
onClick={ () => {
|
||||
onRemove();
|
||||
removeItem();
|
||||
dispatchStoreEvent(
|
||||
'cart-remove-item',
|
||||
{
|
||||
product: lineItem,
|
||||
quantity,
|
||||
}
|
||||
);
|
||||
speak(
|
||||
sprintf(
|
||||
/* translators: %s refers to the item name in the cart. */
|
||||
__(
|
||||
'%s has been removed from your cart.',
|
||||
'woo-gutenberg-products-block'
|
||||
),
|
||||
name
|
||||
)
|
||||
);
|
||||
} }
|
||||
disabled={ isPendingDelete }
|
||||
>
|
||||
{ __(
|
||||
'Remove item',
|
||||
'woo-gutenberg-products-block'
|
||||
) }
|
||||
</button>
|
||||
) }
|
||||
</div>
|
||||
</div>
|
||||
</td>
|
||||
|
|
|
@ -6,6 +6,8 @@ import { previewCart } from '@woocommerce/resource-previews';
|
|||
import { dispatch } from '@wordpress/data';
|
||||
import { CART_STORE_KEY as storeKey } from '@woocommerce/block-data';
|
||||
import { default as fetchMock } from 'jest-fetch-mock';
|
||||
import { __experimentalRegisterCheckoutFilters } from '@woocommerce/blocks-checkout';
|
||||
|
||||
/**
|
||||
* Internal dependencies
|
||||
*/
|
||||
|
@ -230,4 +232,27 @@ describe( 'Testing cart', () => {
|
|||
|
||||
expect( quantityInput.value ).toBe( '5' );
|
||||
} );
|
||||
|
||||
it( 'does not show the remove item button when a filter prevents this', async () => {
|
||||
const cart = {
|
||||
...previewCart,
|
||||
// Make it so there is only one item to simplify things.
|
||||
items: [ previewCart.items[ 0 ] ],
|
||||
};
|
||||
|
||||
__experimentalRegisterCheckoutFilters( 'woo-blocks-test-extension', {
|
||||
showRemoveItemLink: ( value, extensions, { cartItem } ) => {
|
||||
return cartItem.id !== cart.items[ 0 ].id;
|
||||
},
|
||||
} );
|
||||
render( <CartBlock /> );
|
||||
|
||||
await waitFor( () => expect( fetchMock ).toHaveBeenCalled() );
|
||||
|
||||
act( () => {
|
||||
dispatch( storeKey ).receiveCart( cart );
|
||||
} );
|
||||
|
||||
expect( screen.queryAllByText( /Remove item/i ).length ).toBe( 0 );
|
||||
} );
|
||||
} );
|
||||
|
|
|
@ -22,16 +22,17 @@ This document lists the filters that are currently available to extensions and o
|
|||
|
||||
Line items refer to each item listed in the cart or checkout. For instance, the "Sunglasses" and "Beanie with Logo" in this image are the line items.
|
||||
|
||||
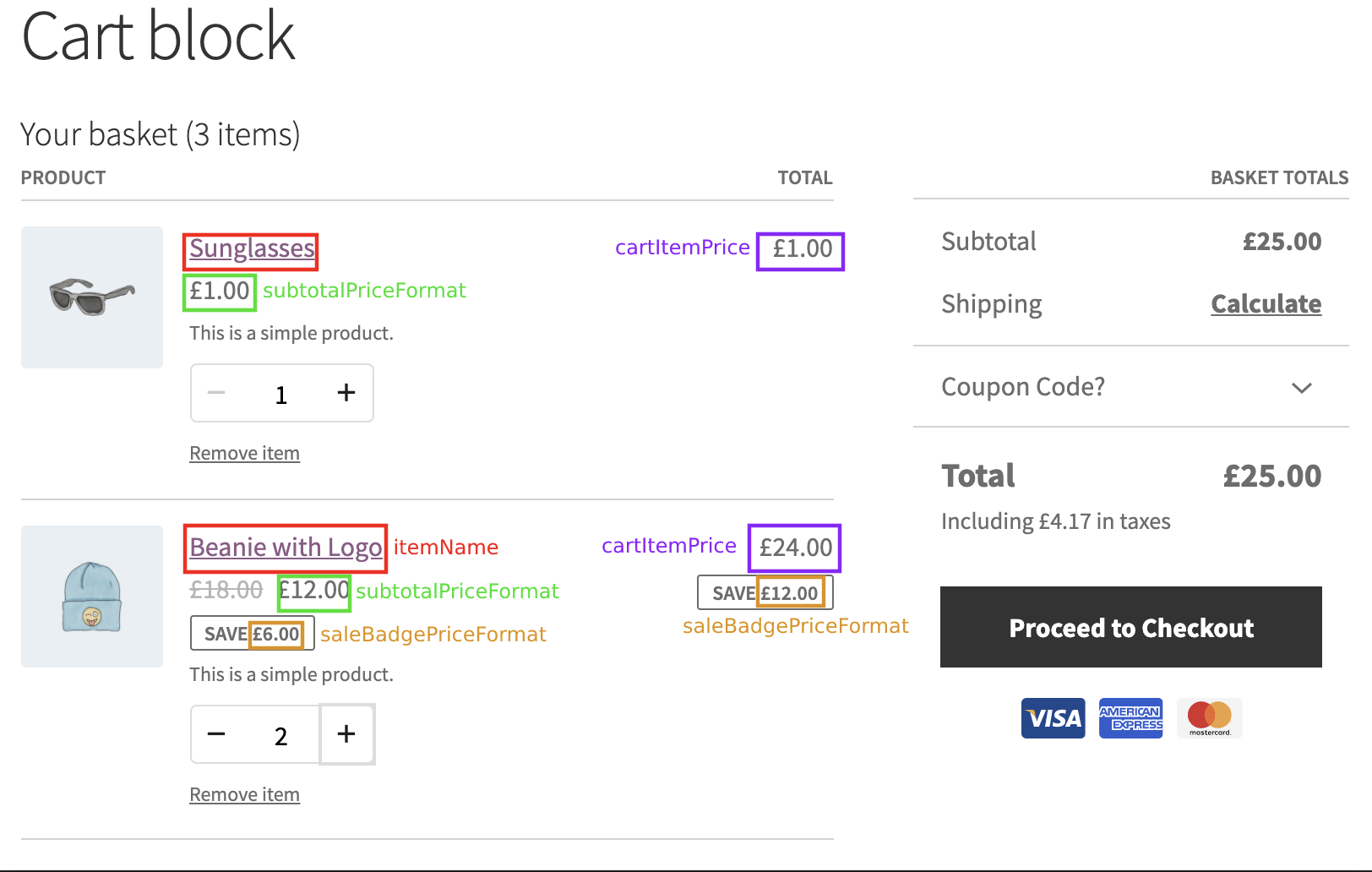
|
||||
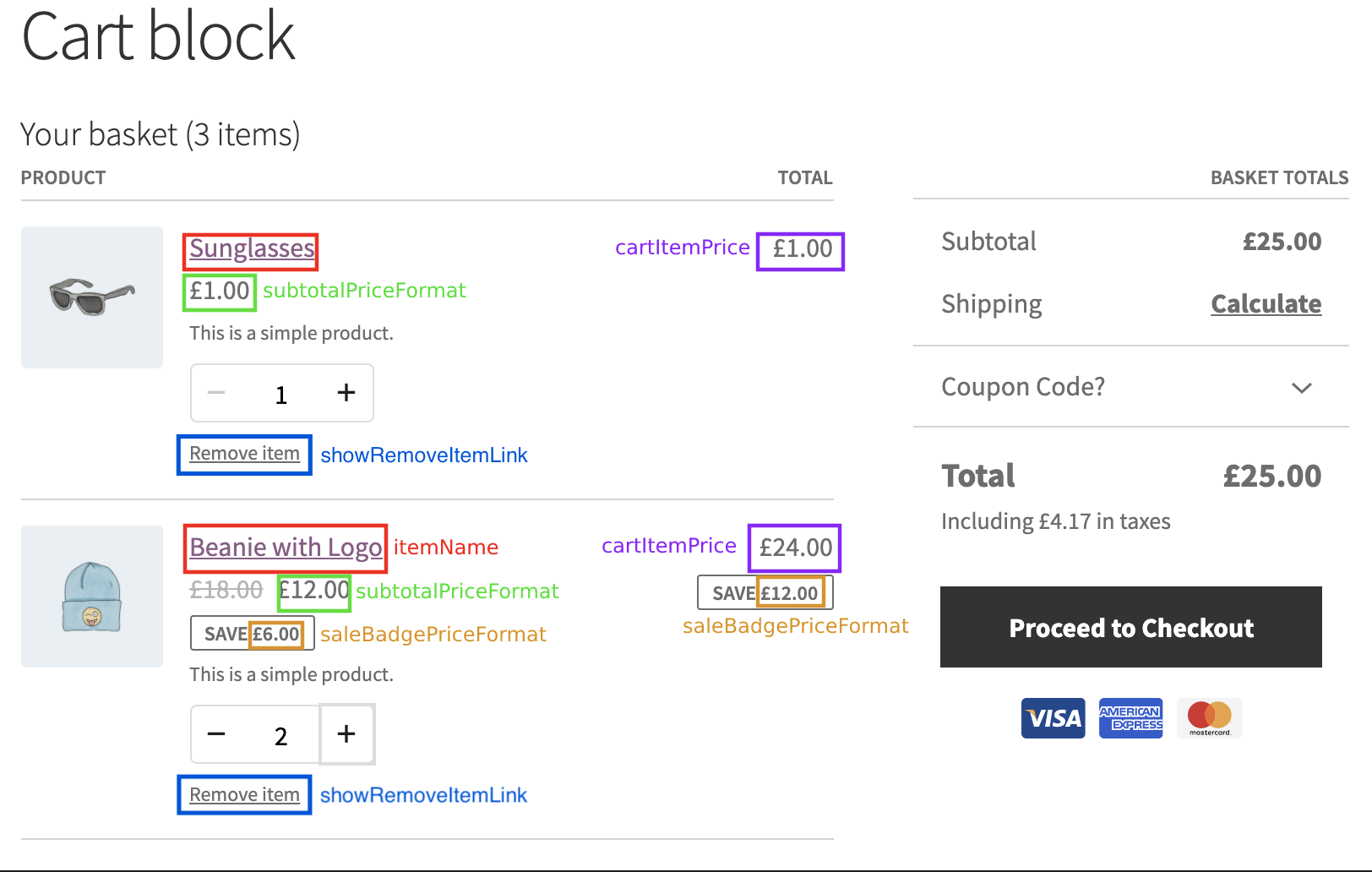
|
||||
|
||||
The following filters are available for line items:
|
||||
|
||||
| Filter name | Description | Return type |
|
||||
| ---------------------- | -------------------------------------------------------------------------------------------------------------------------------------- | ------------------------------------------------------------------------------------- |
|
||||
| ---------------------- |----------------------------------------------------------------------------------------------------------------------------------------|---------------------------------------------------------------------------------------|
|
||||
| `itemName` | Used to change the name of the item before it is rendered onto the page | `string` |
|
||||
| `cartItemPrice` | This is the price of the item, multiplied by the number of items in the cart. | `string` and **must** contain the substring `<price/>` where the price should appear. |
|
||||
| `cartItemClass` | This is the className of the item cell. | `string` |
|
||||
| `subtotalPriceFormat` | This is the price of a single item. Irrespective of the number in the cart, this value will always be the current price of _one_ item. | `string` and **must** contain the substring `<price/>` where the price should appear. |
|
||||
| `showRemoveItemLink` | Toggles the display of the "Remove item" link from the cart line item. Default: `true` | `boolean` |
|
||||
| `saleBadgePriceFormat` | This is the amount of money saved when buying this item. It is the difference between the item's regular price and its sale price. | `string` and **must** contain the substring `<price/>` where the price should appear. |
|
||||
|
||||
Each of these filters has the following arguments passed to it: `{ context: 'cart', cartItem: CartItem }` ([CartItem](https://github.com/woocommerce/woocommerce-gutenberg-products-block/blob/c00da597efe4c16fcf5481c213d8052ec5df3766/assets/js/type-defs/cart.ts#L113))
|
||||
|
@ -230,6 +231,25 @@ __experimentalRegisterCheckoutFilters( 'automatic-coupon-extension', {
|
|||
} );
|
||||
```
|
||||
|
||||
### Hide the "Remove item" link on a cart item
|
||||
|
||||
If you want to stop customers from being able to remove a specific item from their cart **on the front end**, you can do
|
||||
this by using the `showRemoveItemLink` filter. If it returns `false` for that line item the link will not show.
|
||||
|
||||
An important caveat to note is this does _not_ prevent the item from being removed from the cart using StoreAPI or by
|
||||
removing it in the Mini Cart, or traditional shortcode cart.
|
||||
|
||||
```ts
|
||||
import { __experimentalRegisterCheckoutFilters } from '@woocommerce/blocks-checkout';
|
||||
|
||||
__experimentalRegisterCheckoutFilters( 'example-extension', {
|
||||
showRemoveItemLink: ( value, extensions, { cartItem } ) => {
|
||||
// Prevent items with ID 1 being removed from the cart.
|
||||
return cartItem.id !== 1;
|
||||
},
|
||||
} );
|
||||
```
|
||||
|
||||
### Change the label of the Place Order button
|
||||
|
||||
Let's assume a merchant want to change the label of the Place Order button _Place Order_ to _Pay now_. A merchant can achieve this by using the `placeOrderButtonLabel` filter.
|
||||
|
|
Loading…
Reference in New Issue